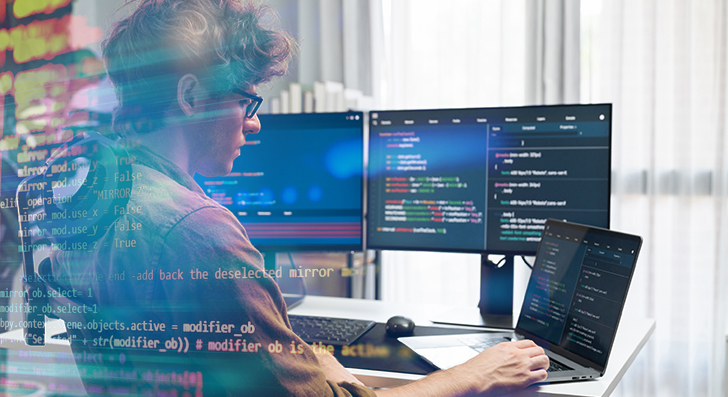
Scalability usually means your software can cope with progress—a lot more customers, extra facts, and a lot more targeted traffic—with out breaking. Being a developer, creating with scalability in your mind saves time and stress later on. Right here’s a transparent and useful guide to assist you start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it should be section of the strategy from the start. Numerous apps fail if they expand speedy since the first design and style can’t deal with the additional load. To be a developer, you should Imagine early about how your process will behave stressed.
Commence by building your architecture to get adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. As a substitute, use modular style or microservices. These designs split your application into more compact, unbiased parts. Each and every module or assistance can scale By itself without affecting The full procedure.
Also, think about your databases from working day one. Will it want to deal with 1,000,000 buyers or just a hundred? Choose the proper style—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
Yet another vital point is to stay away from hardcoding assumptions. Don’t write code that only functions below existing situations. Think of what would come about If the consumer base doubled tomorrow. Would your application crash? Would the databases slow down?
Use layout designs that help scaling, like concept queues or occasion-driven methods. These assist your application cope with additional requests devoid of finding overloaded.
Any time you Make with scalability in your mind, you are not just getting ready for achievement—you might be lessening future problems. A well-prepared process is less complicated to keep up, adapt, and increase. It’s far better to prepare early than to rebuild later on.
Use the proper Databases
Picking out the appropriate database is a vital Section of creating scalable applications. Not all databases are constructed the same, and utilizing the Improper one can gradual you down as well as trigger failures as your application grows.
Start off by comprehending your data. Can it be hugely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely sturdy with relationships, transactions, and consistency. Additionally they support scaling tactics like read replicas, indexing, and partitioning to manage much more targeted visitors and info.
In the event your info is a lot more flexible—like user action logs, products catalogs, or documents—take into account a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with massive volumes of unstructured or semi-structured data and may scale horizontally extra easily.
Also, take into account your read and compose styles. Are you currently undertaking many reads with fewer writes? Use caching and browse replicas. Will you be handling a major create load? Investigate databases which can take care of superior create throughput, as well as party-based info storage programs like Apache Kafka (for short term facts streams).
It’s also good to Believe ahead. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t will need to modify afterwards.
Use indexing to hurry up queries. Avoid unnecessary joins. Normalize or denormalize your information according to your accessibility designs. And constantly keep an eye on databases performance as you grow.
In short, the proper database depends upon your app’s structure, velocity requires, And exactly how you hope it to develop. Consider time to pick correctly—it’ll help save a great deal of difficulties later on.
Optimize Code and Queries
Quick code is key to scalability. As your application grows, just about every small hold off provides up. Badly composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Get started by producing clean up, basic code. Stay away from repeating logic and remove just about anything unwanted. Don’t select the most complex Alternative if an easy 1 is effective. Maintain your functions brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too prolonged to run or works by using a lot of memory.
Following, take a look at your databases queries. These frequently gradual issues down in excess of the code itself. Ensure that Each and every question only asks for the data you really need. Stay clear of Decide on *, which fetches everything, and alternatively select certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically throughout big tables.
In case you recognize the exact same information currently being asked for again and again, use caching. Retailer the final results temporarily making use of instruments like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to examination with massive datasets. Code and queries that do the job fine with one hundred data could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when desired. These steps assist your software continue to be sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to handle more users and much more site visitors. If every little thing goes by means of a single server, it is going to quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app quick, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout many servers. As an alternative to one particular server accomplishing many of the get the job done, the load balancer routes end users to diverse servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused promptly. When consumers request the exact same data once more—like an item webpage or a profile—you don’t really need to fetch it through the database every time. You may serve it in the cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static information near the user.
Caching lowers database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up-to-date when details does transform.
In short, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app cope with much more end users, continue to be quick, and Get well from complications. If you plan to expand, you would like equally.
Use Cloud and Container Applications
To construct scalable programs, you require applications that let your app increase quickly. That’s where cloud platforms and containers come in. They give you versatility, lower set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Internet Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to lease servers and companies as you require them. You don’t really have to buy hardware or guess long term capacity. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or mechanically making use of automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to give attention to developing your app instead of managing infrastructure.
Containers are A further vital Resource. A container deals your app and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of a number of containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it immediately.
Containers also make it very easy to separate aspects of your app into services. You may update or scale elements independently, which is great for performance and trustworthiness.
In brief, utilizing cloud and container instruments indicates you could scale quickly, deploy conveniently, and Recuperate immediately when troubles materialize. If you need your application to expand without the need of limitations, start out utilizing these equipment early. They help you save time, minimize hazard, and enable you to continue to be focused on making, not correcting.
Check Anything
If you don’t keep an eye on your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make greater conclusions as your application grows. It’s a key Portion of building scalable here methods.
Start off by monitoring essential metrics like CPU usage, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application much too. Regulate how much time it takes for users to load pages, how often problems transpire, and wherever they come about. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. One example is, If the reaction time goes earlier mentioned a limit or even a support goes down, you ought to get notified right away. This assists you repair issues fast, normally in advance of users even notice.
Checking is likewise valuable once you make modifications. If you deploy a new element and see a spike in mistakes or slowdowns, you can roll it again just before it leads to serious problems.
As your app grows, traffic and details enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the right instruments in position, you continue to be in control.
To put it briefly, monitoring helps you maintain your app reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your procedure and ensuring it really works nicely, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest apps need to have a solid foundation. By coming up with cautiously, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking under pressure. Commence smaller, Believe massive, and Establish intelligent.